Qoob Portfolio
The open qoob is a php mvc framework created to speed up the process of creating dynamic sites. This is the code running this website!
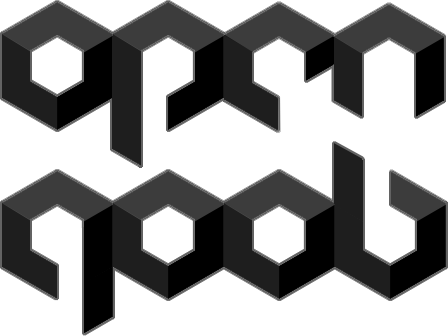
The open qoob is a php mvc framework created to speed up the process of creating dynamic sites. This is the code running this website!
<?php
/**
* portfolio git code browser
*
* @author andrew harrison <andrew@harrison.nu>
* @copyright creative commons attribution-shareAlike 3.0 unported
* @license http://creativecommons.org/licenses/by-sa/3.0/
* @version 0.0.6
*/
namespace app;
class code extends base {
/**
* index
* list repos
*/
function index() {
$this->qoob->load('qoob\core\view\stache');
$content = $this->qoob->stache->render(
'nav',
array(
'domain' => $this->domain,
'code' => 'class="active"'
),
true
);
$this->qoob->load('app\model\code_model');
$repositories = $this->qoob->code_model->repos();
$repos = '';
if(!isset($repositories[0])) {
$repos = '<article><section>No code found...</section></article>';
} else {
foreach ($repositories as $repo) {
//create download link for newest commit on master
$this->git_init($repo['repo']);
$gitcommit = $this->qoob->git->getTip('master');
$obj = $this->qoob->git->getObject($gitcommit);
$hist = $obj->getHistory();
$hist = array_reverse($hist);
$commit = \qoob\utils\sha1_hex($hist[0]->name);
$gitcommit = $this->qoob->git->getObject(\qoob\utils\sha1_bin($commit));
$tree = $gitcommit->getTree();
$url = $this->domain.'/code/'.$repo['url'].'/master/'.$commit;
$dl = $url.'/tree/'.\qoob\utils\sha1_hex($tree->name).'/zip';
//display demo link is available
$view = $repo['demo'] != '' ?
'<li><a href="'.$repo['demo'].'"><i class="fa fa-eye"></i></a></li>' :
'';
$repos .= $this->qoob->stache->render(
'code-repo',
array(
'title' => $repo['name'],
'subtitle' => $repo['subtitle'],
'description' => html_entity_decode($repo['description']),
'clone' => $this->domain.'/repos/'.$repo['repo'],
'download' => $dl,
'url' => $this->domain.'/code/'.$repo['url'],
'view' => $view,
),
true
);
}
}
$content .= $this->qoob->stache->render(
'code-index',
array(
'list' => $repos
),
true
);
$this->qoob->stache->render(
'template',
array(
'author' => \library::get('CONFIG.GENERAL.author'),
'copyright' => \library::get('CONFIG.GENERAL.copyrightHTML'),
'keywords' => \library::get('CONFIG.GENERAL.keywords'),
'description' => \library::get('CONFIG.GENERAL.description'),
'domain' => $this->domain,
'title' => 'personal programming portfolio',
'page' => 'code',
'content' => $content,
'year'=> date('Y'),
)
);
}
/**
* repo
* repository overview
*
* @param array $args url arguments
*/
function repo($args) {
$this->qoob->load('app\model\code_model');
$repo = $this->qoob->code_model->get_repo(
isset($args['repo']) ?
$args['repo'] :
''
);
if(!isset($repo[0])) {
throw new \Exception("Unknown git repo", 404);
}
$this->git_init($repo[0]['repo']);
$branch = 'master';
$this->qoob->load('qoob\core\view\stache');
$content = $this->qoob->stache->render(
'nav',
array(
'domain' => $this->domain,
'code' => 'class="active"'
),
true
);
$image = $repo[0]['preview'] != '' ?
'<img src="'.$this->domain.'/ui/img/code/'.$repo[0]['preview'].'" alt="'.$repo[0]['name'].'" />' :
'';
$url = $this->domain.'/code/'.$repo[0]['url'];
$content .= $this->qoob->stache->render(
'code-repo-header',
array(
'title' => $repo[0]['name'],
'subtitle' => $repo[0]['subtitle'],
'image' => $image,
'clone' => $this->domain.'/code/'.$repo[0]['repo'],
'history' => $this->domain.'/code/'.$repo[0]['url'].'/'.$branch.'/history',
'branches' => $this->git_branches($url, $branch),
'commits' => $this->git_commits($url, $branch, '')
),
true
);
$content .= $this->qoob->stache->render(
'code-repo-files',
$this->git_tree($repo[0]['url'], $branch, ''),
true
);
$this->qoob->stache->render(
'template',
array(
'author' => \library::get('CONFIG.GENERAL.author'),
'copyright' => \library::get('CONFIG.GENERAL.copyrightHTML'),
'keywords' => \library::get('CONFIG.GENERAL.keywords'),
'description' => \library::get('CONFIG.GENERAL.description'),
'domain' => $this->domain,
'title' => 'personal programming portfolio',
'page' => 'code',
'content' => $content,
'year'=> date('Y'),
)
);
}
/**
* branch
* git branch overview
*
* @param array $args url arguments
*/
function branch($args) {
$this->qoob->load('app\model\code_model');
$repo = $this->qoob->code_model->get_repo(
isset($args['repo']) ?
$args['repo'] :
''
);
if(!isset($repo[0])) {
throw new \Exception("Unknown git repo", 404);
}
$this->git_init($repo[0]['repo']);
$branch = isset($args['branch']) ? $args['branch'] : '';
if(!in_array($branch, $this->qoob->git->branches)) {
throw new \Exception("Unknown branch", 404);
}
$this->qoob->load('qoob\core\view\stache');
$content = $this->qoob->stache->render(
'nav',
array(
'domain' => $this->domain,
'code' => 'class="active"'
),
true
);
$image = $repo[0]['preview'] != '' ?
'<img src="'.$this->domain.'/ui/img/code/'.$repo[0]['preview'].'" alt="'.$repo[0]['name'].'" />' :
'';
$url = $this->domain.'/code/'.$repo[0]['url'];
$content .= $this->qoob->stache->render(
'code-repo-header',
array(
'title' => $repo[0]['name'],
'subtitle' => $repo[0]['subtitle'],
'image' => $image,
'clone' => $this->domain.'/code/'.$repo[0]['repo'],
'history' => $this->domain.'/code/'.$repo[0]['url'].'/'.$branch.'/history',
'branches' => $this->git_branches($url, $branch),
'commits' => $this->git_commits($url, $branch, '')
),
true
);
$content .= $this->qoob->stache->render(
'code-repo-files',
$this->git_tree($repo[0]['url'], $branch, ''),
true
);
$this->qoob->stache->render(
'template',
array(
'author' => \library::get('CONFIG.GENERAL.author'),
'copyright' => \library::get('CONFIG.GENERAL.copyrightHTML'),
'keywords' => \library::get('CONFIG.GENERAL.keywords'),
'description' => \library::get('CONFIG.GENERAL.description'),
'domain' => $this->domain,
'title' => 'personal programming portfolio',
'page' => 'code',
'content' => $content,
'year'=> date('Y'),
)
);
}
/**
* history
* git repo commit history
*
* @param array $args url arguments
*/
function history($args) {
$this->qoob->load('app\model\code_model');
$repo = $this->qoob->code_model->get_repo(
isset($args['repo']) ?
$args['repo'] :
''
);
if(!isset($repo[0])) {
throw new \Exception("Unknown git repo", 404);
}
$this->git_init($repo[0]['repo']);
$branch = isset($args['branch']) ? $args['branch'] : '';
if(!in_array($branch, $this->qoob->git->branches)) {
throw new \Exception("Unknown branch", 404);
}
$this->qoob->load('qoob\core\view\stache');
$content = $this->qoob->stache->render(
'nav',
array(
'domain' => $this->domain,
'code' => 'class="active"'
),
true
);
$image = $repo[0]['preview'] != '' ?
'<img src="'.$this->domain.'/ui/img/code/'.$repo[0]['preview'].'" alt="'.$repo[0]['name'].'" />' :
'';
$url = $this->domain.'/code/'.$repo[0]['url'];
$content .= $this->qoob->stache->render(
'code-repo-header',
array(
'title' => $repo[0]['name'],
'subtitle' => $repo[0]['subtitle'],
'image' => $image,
'clone' => $this->domain.'/code/'.$repo[0]['repo'],
'history' => $this->domain.'/code/'.$repo[0]['url'].'/'.$branch.'/history',
'branches' => $this->git_branches($url, $branch),
'commits' => $this->git_commits($url, $branch, '')
),
true
);
$gitcommit = $this->qoob->git->getTip($branch);
$obj = $this->qoob->git->getObject($gitcommit);
$hist = $obj->getHistory();
$hist = array_reverse($hist);
$commits = '';
foreach ($hist as $event) {
//just incase the committer info is blank
$name = $event->committer->name === '' ? 'andrew harrison' : $event->committer->name;
$email = $event->committer->email == '' ? 'andrew@harrison.nu' : $event->committer->email;
$commits .= '<li><cite>'.$name.' <'.$email.'><cite><em>'.date('m/d/y', $event->committer->time).'</em><blockquote><a href="'.$this->domain.'/code/'.$repo[0]['url'].'/'.$branch.'/'.\qoob\utils\sha1_hex($event->name).'"><i class="fa fa-code-fork"></i>'.substr(\qoob\utils\sha1_hex($event->name), 0, 10).'</a> '.wordwrap($event->summary, 90).'</blockquote></li>';
}
$content .= $this->qoob->stache->render(
'code-repo-history',
array(
'commits' => $commits
),
true
);
$this->qoob->stache->render(
'template',
array(
'author' => \library::get('CONFIG.GENERAL.author'),
'copyright' => \library::get('CONFIG.GENERAL.copyrightHTML'),
'keywords' => \library::get('CONFIG.GENERAL.keywords'),
'description' => \library::get('CONFIG.GENERAL.description'),
'domain' => $this->domain,
'title' => 'personal programming portfolio',
'page' => 'code',
'content' => $content,
'year'=> date('Y'),
)
);
}
/**
* commit
* git commit overview
*
* @param array $args url arguments
*/
function commit($args) {
$this->qoob->load('app\model\code_model');
$repo = $this->qoob->code_model->get_repo(
isset($args['repo']) ?
$args['repo'] :
''
);
if(!isset($repo[0])) {
throw new \Exception("Unknown git repo", 404);
}
$this->git_init($repo[0]['repo']);
$branch = isset($args['branch']) ? $args['branch'] : '';
$commit = isset($args['commit']) ? $args['commit'] : '';
if(!in_array($branch, $this->qoob->git->branches)) {
throw new \Exception("Unknown branch", 404);
}
$this->qoob->load('qoob\core\view\stache');
$content = $this->qoob->stache->render(
'nav',
array(
'domain' => $this->domain,
'code' => 'class="active"'
),
true
);
$image = $repo[0]['preview'] != '' ?
'<img src="'.$this->domain.'/ui/img/code/'.$repo[0]['preview'].'" alt="'.$repo[0]['name'].'" />' :
'';
$url = $this->domain.'/code/'.$repo[0]['url'];
$content .= $this->qoob->stache->render(
'code-repo-header',
array(
'title' => $repo[0]['name'],
'subtitle' => $repo[0]['subtitle'],
'image' => $image,
'clone' => $this->domain.'/code/'.$repo[0]['repo'],
'history' => $this->domain.'/code/'.$repo[0]['url'].'/'.$branch.'/history',
'branches' => $this->git_branches($url, $branch),
'commits' => $this->git_commits($url, $branch, $commit)
),
true
);
$content .= $this->qoob->stache->render(
'code-repo-files',
$this->git_tree($repo[0]['url'], $branch, $commit),
true
);
$this->qoob->stache->render(
'template',
array(
'author' => \library::get('CONFIG.GENERAL.author'),
'copyright' => \library::get('CONFIG.GENERAL.copyrightHTML'),
'keywords' => \library::get('CONFIG.GENERAL.keywords'),
'description' => \library::get('CONFIG.GENERAL.description'),
'domain' => $this->domain,
'title' => 'personal programming portfolio',
'page' => 'code',
'content' => $content,
'year'=> date('Y'),
)
);
}
/**
* tree
* git commit tree
*
* @param array $args url arguments
*/
function tree($args) {
$this->qoob->load('app\model\code_model');
$repo = $this->qoob->code_model->get_repo(
isset($args['repo']) ?
$args['repo'] :
''
);
if(!isset($repo[0])) {
throw new \Exception("Unknown git repo", 404);
}
$this->git_init($repo[0]['repo']);
$branch = isset($args['branch']) ? $args['branch'] : '';
$commit = isset($args['commit']) ? $args['commit'] : '';
$obj = isset($args['obj']) ? $args['obj'] : '';
if(!in_array($branch, $this->qoob->git->branches)) {
throw new \Exception("Unknown branch", 404);
}
$this->qoob->load('qoob\core\view\stache');
$content = $this->qoob->stache->render(
'nav',
array(
'domain' => $this->domain,
'code' => 'class="active"'
),
true
);
$image = $repo[0]['preview'] != '' ?
'<img src="'.$this->domain.'/ui/img/code/'.$repo[0]['preview'].'" alt="'.$repo[0]['name'].'" />' :
'';
$url = $this->domain.'/code/'.$repo[0]['url'];
$content .= $this->qoob->stache->render(
'code-repo-header',
array(
'title' => $repo[0]['name'],
'subtitle' => $repo[0]['subtitle'],
'image' => $image,
'clone' => $this->domain.'/code/'.$repo[0]['repo'],
'history' => $this->domain.'/code/'.$repo[0]['url'].'/'.$branch.'/history',
'branches' => $this->git_branches($url, $branch),
'commits' => $this->git_commits($url, $branch, $commit)
),
true
);
$content .= $this->qoob->stache->render(
'code-repo-files',
$this->git_tree($repo[0]['url'], $branch, $commit, $obj),
true
);
$this->qoob->stache->render(
'template',
array(
'author' => \library::get('CONFIG.GENERAL.author'),
'copyright' => \library::get('CONFIG.GENERAL.copyrightHTML'),
'keywords' => \library::get('CONFIG.GENERAL.keywords'),
'description' => \library::get('CONFIG.GENERAL.description'),
'domain' => $this->domain,
'title' => 'personal programming portfolio',
'page' => 'code',
'content' => $content,
'year'=> date('Y'),
)
);
}
/**
* blob
* git commit blob data
*
* @param array $args url arguments
*/
function blob($args) {
$this->qoob->load('app\model\code_model');
$repo = $this->qoob->code_model->get_repo(
isset($args['repo']) ?
$args['repo'] :
''
);
if(!isset($repo[0])) {
throw new \Exception("Unknown git repo", 404);
}
$this->git_init($repo[0]['repo']);
$branch = isset($args['branch']) ? $args['branch'] : '';
$commit = isset($args['commit']) ? $args['commit'] : '';
$obj = isset($args['obj']) ? $args['obj'] : '';
if(!in_array($branch, $this->qoob->git->branches)) {
throw new \Exception("Unknown branch", 404);
}
$this->qoob->load('qoob\core\view\stache');
$content = $this->qoob->stache->render(
'nav',
array(
'domain' => $this->domain,
'code' => 'class="active"'
),
true
);
$image = $repo[0]['preview'] != '' ?
'<img src="'.$this->domain.'/ui/img/code/'.$repo[0]['preview'].'" alt="'.$repo[0]['name'].'" />' :
'';
$url = $this->domain.'/code/'.$repo[0]['url'];
$content .= $this->qoob->stache->render(
'code-repo-header',
array(
'title' => $repo[0]['name'],
'subtitle' => $repo[0]['subtitle'],
'image' => $image,
'clone' => $this->domain.'/code/'.$repo[0]['repo'],
'history' => $this->domain.'/code/'.$repo[0]['url'].'/'.$branch.'/history',
'branches' => $this->git_branches($url, $branch),
'commits' => $this->git_commits($url, $branch, $commit)
),
true
);
$content .= $this->qoob->stache->render(
'code-repo-blob',
$this->git_blob(false, $commit, $obj, $this->domain.'/code/'.$repo[0]['url'].'/'.$branch.'/'.$commit.'/blob/'.$obj),
true
);
$this->qoob->stache->render(
'template',
array(
'author' => \library::get('CONFIG.GENERAL.author'),
'copyright' => \library::get('CONFIG.GENERAL.copyrightHTML'),
'keywords' => \library::get('CONFIG.GENERAL.keywords'),
'description' => \library::get('CONFIG.GENERAL.description'),
'domain' => $this->domain,
'title' => 'personal programming portfolio',
'page' => 'code',
'content' => $content,
'year'=> date('Y'),
)
);
}
/**
* raw
* git commit raw blob data
*
* @param array $args url arguments
*/
function raw($args) {
$this->qoob->load('app\model\code_model');
$repo = $this->qoob->code_model->get_repo(
isset($args['repo']) ?
$args['repo'] :
''
);
if(!isset($repo[0])) {
throw new \Exception("Unknown git repo", 404);
}
$this->git_init($repo[0]['repo']);
$branch = isset($args['branch']) ? $args['branch'] : '';
$commit = isset($args['commit']) ? $args['commit'] : '';
$obj = isset($args['obj']) ? $args['obj'] : '';
if(!in_array($branch, $this->qoob->git->branches)) {
throw new \Exception("Unknown branch", 404);
}
$this->qoob->load('qoob\core\view\stache');
$blob = $this->git_blob(true, $commit, $obj, $this->domain.'/code/'.$repo[0]['url'].'/'.$branch.'/blob/'.$obj);
if (PHP_SAPI!='cli') {
switch($blob['type'] ){
case 'txt':
header('Content-Type: text/plain; charset=utf-8');
echo html_entity_decode($blob['blob']);
break;
case 'img':
switch ($blob['ext']) {
case 'jpg':
header('Content-Type: image/jpeg');
break;
case 'jpeg':
header('Content-Type: image/jpeg');
break;
case 'png':
header('Content-Type: image/png');
break;
case 'gif':
header('Content-Type: image/gif');
break;
case 'bmp':
header('Content-Type: image/bmp');
break;
case 'ico':
header('Content-Type: image/ico');
break;
case 'svg':
header('Content-Type: image/svg+xml');
break;
}
$img = imagecreatefromstring($blob['blob']);
imagepng($img);
imagedestroy($img);
break;
default:
header('Content-Disposition: attachment; filename="'.substr(strrchr($blob['file'],'/'),1).'"');
echo $blob['blob'];
break;
}
}
return;
}
/**
* export
* git commit export zip
*
* @param array $args url arguments
*/
function zip($args) {
$this->qoob->load('app\model\code_model');
$repo = $this->qoob->code_model->get_repo(
isset($args['repo']) ?
$args['repo'] :
''
);
if(!isset($repo[0])) {
throw new \Exception("Unknown git repo", 404);
}
$this->git_init($repo[0]['repo']);
$branch = isset($args['branch']) ? $args['branch'] : '';
$obj = isset($args['obj']) ? $args['obj'] : '';
if(!in_array($branch, $this->qoob->git->branches)) {
throw new \Exception("Unknown branch", 404);
}
$archive = $this->qoob->git->archive($repo[0]['repo'].'-'.substr($obj, 0, 7), \library::get('QOOB.root').'/repos/'.$repo[0]['repo'], $obj, 'zip');
echo $archive;
return;
}
/**
* tar export
* git commit export tarball
*
* @param array $args url arguments
*/
function tar($args) {
$this->qoob->load('app\model\code_model');
$repo = $this->qoob->code_model->get_repo(
isset($args['repo']) ?
$args['repo'] :
''
);
if(!isset($repo[0])) {
throw new \Exception("Unknown git repo", 404);
}
$this->git_init($repo[0]['repo']);
$branch = isset($args['branch']) ? $args['branch'] : '';
$obj = isset($args['obj']) ? $args['obj'] : '';
if(!in_array($branch, $this->qoob->git->branches)) {
throw new \Exception("Unknown branch", 404);
}
$archive = $this->qoob->git->archive($repo[0]['repo'].'-'.substr($obj, 0, 7), \library::get('QOOB.root').'/repos/'.$repo[0]['repo'], $obj, 'tar');
echo $archive;
return;
}
//___________________________________________________________________
// git functions
private function git_init($repo) {
$this->qoob->load('qoob\utils\git');
$this->qoob->git->init(\library::get('QOOB.root').'/repos/'.$repo);
}
private function git_branches($url, $branch) {
$branches = '';
foreach ($this->qoob->git->branches as $theBranch) {
if($theBranch == $branch) {
$branches .= '<option value="'.$url.'/'.$theBranch.'" selected="selected">'.$theBranch.'</option>';
} else {
$branches .= '<option value="'.$url.'/'.$theBranch.'">'.$theBranch.'</option>';
}
}
return $branches;
}
private function git_commits($url, $branch, $commit){
$gitcommit = $this->qoob->git->getTip($branch);
$obj = $this->qoob->git->getObject($gitcommit);
$hist = $obj->getHistory();
$hist = array_reverse($hist);
$commits = '';
foreach ($hist as $event) {
if(\qoob\utils\sha1_hex($event->name) == $commit) {
$commits .= '<option value="'.$url.'/'.$branch.'/'.\qoob\utils\sha1_hex($event->name).'" selected="selected">'.date('m/d/y g:ia', $event->committer->time).'</option>';
} else {
$commits .= '<option value="'.$url.'/'.$branch.'/'.\qoob\utils\sha1_hex($event->name).'">'.date('m/d/y g:ia', $event->committer->time).'</option>';
}
}
return $commits;
}
private function git_tree($repo, $branch, $commit = '', $objid = ''){
if($commit === '') {
$gitcommit = $this->qoob->git->getTip($branch);
$obj = $this->qoob->git->getObject($gitcommit);
$hist = $obj->getHistory();
$hist = array_reverse($hist);
$commit = \qoob\utils\sha1_hex($hist[0]->name);
}
if($objid === '') {
$gitcommit = $this->qoob->git->getObject(\qoob\utils\sha1_bin($commit));
$tree = $gitcommit->getTree();
} else {
$tree = $this->qoob->git->getObject(\qoob\utils\sha1_bin($objid));
}
$url = $this->domain.'/code/'.$repo.'/'.$branch.'/'.$commit;
$dl = $url.'/tree/'.\qoob\utils\sha1_hex($tree->name);
$files = '';
foreach ($tree->nodes as $file) {
if($file->is_dir) {
$files .= '<li><i class="fa fa-li fa-folder"></i><a href="'.$url.'/tree/'.\qoob\utils\sha1_hex($file->object).'">'.$file->name.'</a></li>';
}
}
foreach ($tree->nodes as $file) {
if(!$file->is_dir) {
$files .= '<li><i class="fa fa-li fa-file"></i><a href="'.$url.'/blob/'.\qoob\utils\sha1_hex($file->object).'">'.$file->name.'</a></li>';
}
}
return array(
'tree' => $files,
'download' => $dl
);
}
private function git_blob($raw, $commit, $objid, $url) {
$blob = $this->qoob->git->getObject(\qoob\utils\sha1_bin($objid));
$gitcommit = $this->qoob->git->getObject(\qoob\utils\sha1_bin($commit));
$tree = $gitcommit->getTree()->listRecursive();
$file = '';
$ext = '';
$type = 'unknown';
foreach ($tree as $name => $sha) {
if(\qoob\utils\sha1_hex($sha) == $objid) {
$file = $name;
$ext = substr(strrchr($name,'.'),1);
}
}
if(in_array($ext, array(
'png',
'jpg',
'gif',
'bmp',
'ico',
'svg'
))){
$type = 'img';
}
if(in_array($ext, array(
'txt',
'html',
'xml',
'json',
'css',
'scss',
'js',
'php',
'mxml',
'as',
'sql',
'hbs',
'handlebars',
'log',
'dat',
'md'
))){
$type = 'txt';
}
if(strpos($file, '.') === 0){
$type = 'txt';
}
if(
strpos(strtolower($file), 'read_me') != false ||
strpos(strtolower($file), 'readme') != false ||
strpos(strtolower($file), 'read.me') != false ||
strpos(strtolower($file), 'read-me') != false
){
$type = 'txt';
}
$data = $blob->data;
if(!$raw) {
if($type == 'img') {
switch ($ext) {
case 'jpg':
$data = '<img src="data:image/jpeg;base64,'.base64_encode($blob->data).'" alt="'.$file.'"/>';
break;
case 'jpeg':
$data = '<img src="data:image/jpeg;base64,'.base64_encode($blob->data).'" alt="'.$file.'"/>';
break;
case 'png':
$data = '<img src="data:image/png;base64,'.base64_encode($blob->data).'" alt="'.$file.'"/>';
break;
case 'gif':
$data = '<img src="data:image/gif;base64,'.base64_encode($blob->data).'" alt="'.$file.'"/>';
break;
case 'bmp':
$data = '<img src="data:image/bmp;base64,'.base64_encode($blob->data).'" alt="'.$file.'"/>';
break;
case 'ico':
$data = '<img src="data:image/bmp;base64,'.base64_encode($blob->data).'" alt="'.$file.'"/>';
break;
case 'svg':
$data = $blob->data;
break;
default:
$data = '<p>This image is not correctly formatted, so cannot be displayed. sorry :(<br/>press the raw button below to download it or clone the git repo to get a copy.</p>';
break;
}
} else if($type == 'txt') {
$data = '<pre><code>'.htmlentities($blob->data).'</code></pre>';
} else {
$data = '<p>This data is not ascii formatted, so cannot be displayed. sorry :(<br/>press the raw button below to download it or clone the git repo to get a copy.</p>';
}
}
return array(
'blob' => $data,
'type' => $type,
'file' => $file,
'ext' => $ext,
'url' => $url
);
}
}
?>