Lorenz Attractor
Plotting chaotic behavior in 3D
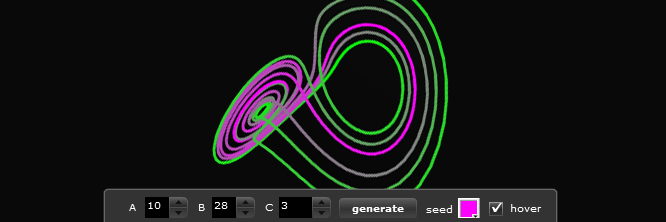
Plotting chaotic behavior in 3D
package com.fontvirus
{
import flash.display.*;
import flash.events.*;
import flash.text.*;
import mx.core.UIComponent;
public class FlexFS extends UIComponent
{
private var theBox :Sprite = new Sprite();
private var theAni :Sprite = new Sprite();
private var theHit :Sprite = new Sprite();
private var pos :String;
private var base :uint;
private var line :uint;
private var xpos :Number = 0;
private var ypos :Number = 0;
private var level :Number = 0;
private var isMoving :Boolean = false;
private var expand :Boolean = true;
private var goFull :Boolean = false;
public function FlexFS(basecolor:uint = 0x000000, linecolor:uint = 0xcccccc, position:String = "topLeft", maxX:Number = 0, maxY:Number=0)
{
base = basecolor;
line = linecolor;
pos = position;
setup(maxX, maxY);
theHit.buttonMode = true;
theHit.addEventListener(MouseEvent.MOUSE_OVER, mouseOver);
theHit.addEventListener(MouseEvent.MOUSE_OUT, mouseOut);
theHit.addEventListener(MouseEvent.CLICK, mouseClick);
this.addEventListener(Event.ENTER_FRAME, loop);
}
private function loop(e:Event):void
{
if(isMoving){
if(expand){
level++;
} else {
level--;
}
if(level==0){
theAni.graphics.clear();
theAni.graphics.beginFill(base, 1);
theAni.graphics.lineStyle(1, line, 1);
theAni.graphics.drawRect(xpos+30, ypos+20, 100, 50);
if(!expand){
isMoving = false;
}
} else if(level==2) {
theAni.graphics.clear();
theAni.graphics.beginFill(base, 1);
theAni.graphics.lineStyle(1, line, 1);
theAni.graphics.drawRect(xpos+25, ypos+18, 110, 60);
} else if(level==4) {
theAni.graphics.clear();
theAni.graphics.beginFill(base, 1);
theAni.graphics.lineStyle(1, line, 1);
theAni.graphics.drawRect(xpos+20, ypos+15, 120, 70);
} else if(level==6) {
theAni.graphics.clear();
theAni.graphics.beginFill(base, 1);
theAni.graphics.lineStyle(1, line, 1);
theAni.graphics.drawRect(xpos+15, ypos+10, 130, 75);
} else if(level==8) {
theAni.graphics.clear();
theAni.graphics.beginFill(base, 1);
theAni.graphics.lineStyle(1, line, 1);
theAni.graphics.drawRect(xpos+10, ypos+5, 141, 80);
} else if(level==10) {
theAni.graphics.clear();
theAni.graphics.beginFill(base, 1);
theAni.graphics.lineStyle(1, line, 1);
theAni.graphics.drawRect(xpos+5, ypos+3, 151, 85);
} else if(level==12) {
if(expand){
isMoving = false;
}
theAni.graphics.clear();
theAni.graphics.beginFill(base, 1);
theAni.graphics.lineStyle(1, line, 1);
theAni.graphics.drawRect(xpos+0, ypos+0, 162, 90);
}
}
}
//---mouse eventz
private function mouseOver(e:Event):void
{
isMoving = true;
if(!goFull) {
expand = true;
level=0;
} else {
expand = false;
level=12;
}
}
private function mouseOut(e:Event):void
{
isMoving = true;
if(!goFull) {
expand = false;
level=12;
} else {
expand = true;
level=0;
}
}
private function mouseClick(e:MouseEvent):void
{
if(goFull)
disableFullScreen()
else
enableFullScreen()
}
//---fullscreen functionz
private function fullScreenRedraw(event:FullScreenEvent):void
{
if (event.fullScreen == false)
disableFullScreen()
}
private function disableFullScreen():void
{
goFull = false;
this.stage.displayState = StageDisplayState.NORMAL;
}
private function enableFullScreen():void
{
goFull = true;
this.stage.displayState = StageDisplayState.FULL_SCREEN;
}
//---resize handler
public function setup(maxX:Number, maxY:Number):void
{
if(pos=="topLeft"){
xpos = 10;
ypos = 10;
} else if(pos=="topRight") {
xpos = (maxX*2) - 190;
ypos = 10;
} else if(pos=="bottomLeft") {
xpos = 10;
ypos = (maxY*2) - 110;
} else if(pos=="bottomRight") {
xpos = (maxX*2) - 190;
ypos = (maxY*2) - 110;
}
theBox.graphics.clear();
theBox.graphics.beginFill(base, 1);
theBox.graphics.lineStyle(1, line, 1);
theBox.graphics.drawRect(xpos, ypos, 162, 90);
theBox.graphics.endFill();
this.addChild(theBox);
theAni.graphics.clear();
theAni.graphics.beginFill(base, 1);
theAni.graphics.lineStyle(1, line, 1);
theAni.graphics.drawRect(xpos+30, ypos+20, 100, 50);
this.addChild(theAni);
theHit.graphics.clear();
theHit.graphics.beginFill(0x000000, 0.001);
theHit.graphics.drawRect(xpos, ypos, 162, 90);
theHit.graphics.endFill();
this.addChild(theHit);
this.scaleX = .5;
this.scaleY = .5;
}
}
}